Before
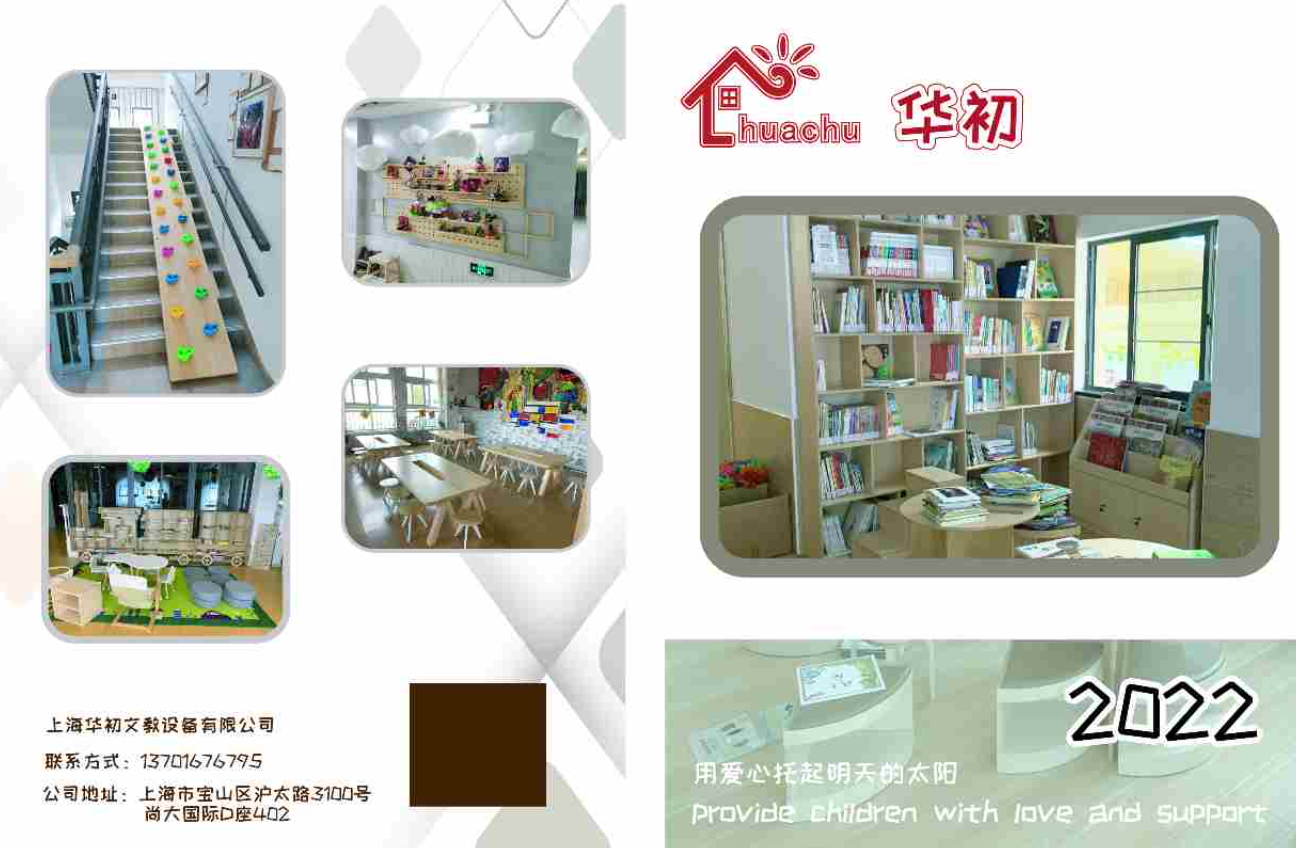
After
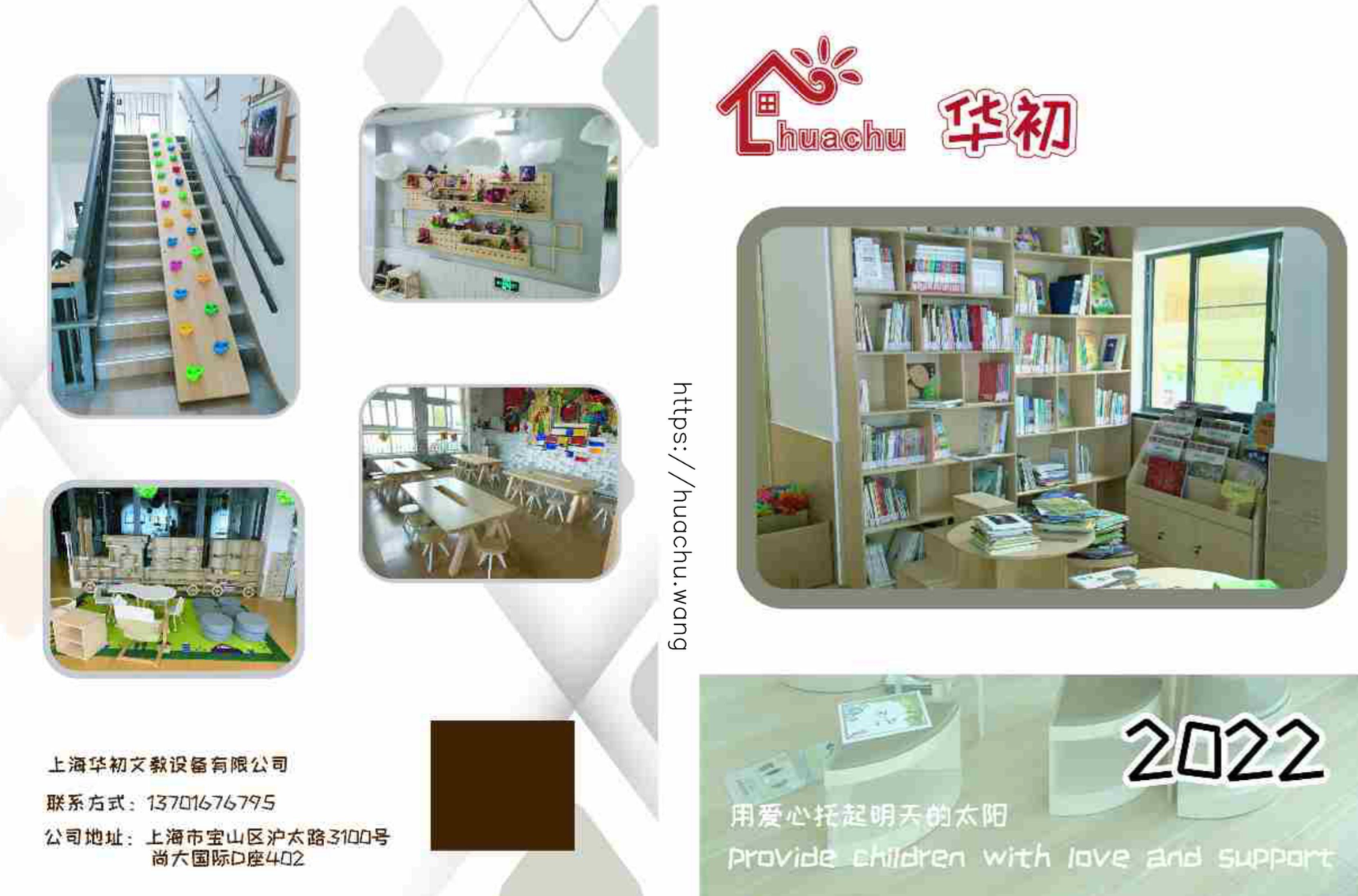
This function can be divided into many steps.
Step 1: turn PDF to image
Step 2: rotate image by 90 degrees
Step 3: add the text in the middle of the image
Step 4: rotate the image back and save
Step 5: merge all images into a PDF
We need following dependencies: cv2, PIL, and pdf2image
The whole code is as follows:
import os, cv2
from tqdm import tqdm
from pdf2image import convert_from_path
from PIL import Image
#############################
# Convert PDF to JPG
#############################
def conver_pdf_to_image():
images = convert_from_path('华初文教.pdf')
for i in range(len(images)):
# Save pages as images in the pdf
images[i].save('page'+ str(i) +'.jpg', 'JPEG')
#############################
# Add the website to JPGs
#############################
def add_website(filename):
# assert
if not os.path.isfile(filename):
print(filename + " doesn't exist")
return
# get image information
img = cv2.imread(filename, cv2.IMREAD_UNCHANGED)
# rotate image
new_img = cv2.rotate(img, cv2.cv2.ROTATE_90_COUNTERCLOCKWISE)
# 2 - add text
msg = "https://huachu.wang"
# font
font = cv2.FONT_HERSHEY_SIMPLEX
# font size
fontScale = 2
# font color - black
fontColor = (20, 20, 20)
# line thickness
fontThickness = 2
# get boundary of this text
textsize = cv2.getTextSize(msg, font, 1, 2)[0]
# get coords based on boundary
textX = int((new_img.shape[1] - textsize[0]) / 2)
textY = int((new_img.shape[0] + textsize[1]) / 2)
# org
org = (textX, textY)
cv2.putText(new_img, msg, org, font, fontScale, fontColor, fontThickness, cv2.LINE_AA)
# rotate the image back
img = cv2.rotate(new_img, cv2.cv2.ROTATE_90_CLOCKWISE)
cv2.imwrite(filename, img)
def merge_pdf():
images = [
Image.open("/Users/dph/Documents/work-company/clients/huachu/material/page"+str(i)+".jpg")
for i in range(0, 87)
]
pdf_path = "result.pdf"
images[0].save(
pdf_path, "PDF", resolution=100.0, save_all=True, append_images=images[1:]
)