When Spring-Cloud-Config Server is enabled, the URL of https://{hostname}:{port}/application/{profile} is accessible.
Occasionally, our configuration files contain sensitive data, prompting the implementation of additional security measures to safeguard them.
I’ve employed the following methods.
- SSL
- Private Github Repo
- Spring Cloud Config enable /encrypt & /decrypt
- Testing on Postman
- How to encrypt the data
- Decrypt on Client side
- Spring Security
SSL
Initially, I implemented SSL configuration to ensure that transportation occurs over HTTPS.
###################
## SSL
###################
server.ssl.enabled=true
server.ssl.key-store-type=PKCS12
server.ssl.key-store=
server.ssl.key-store-password=
trust.store =
trust.store.password=
For guidance on generating the key-store file, you can refer to my other article.
Private Github Repo
Then I modified the Github repository linked to the Spring-Cloud-Config Server, switching it from a public to a private repository to prevent others from obtaining information through the public repository.
spring.cloud.config.server.git.uri=
spring.cloud.config.server.git.username=
spring.cloud.config.server.git.password={Github Personal Access Token}
spring.cloud.config.server.git.default-label=main
/encrypt & /decrypt
Thirdly, I proceeded to encrypt the information accessed from the URL https://{host}:{port}/application/{profile} to prevent access to sensitive data from this endpoint.
management.endpoints.web.exposure.include=*
encrypt.key={Symmetric Key}
This configuration will activate the /encrypt and /decrypt endpoints, requiring implementation in both the Spring-Cloud-Config Server and Client for functionality. Server is responsible for encrypt and Client is for decrypt.
There are two encryption methods available: symmetric and asymmetric key. I suggest using symmetric key at first as it’s simpler and facilitates quicker testing to confirm the successful configuration.
Testing on Postman
For testing purposes, you can utilize POST requests on Postman to the URLs http://localhost:8888/encrypt and http://localhost:8888/decrypt, assuming the host is localhost and the port is 8888. This allows you to verify if these endpoints have been properly set up.
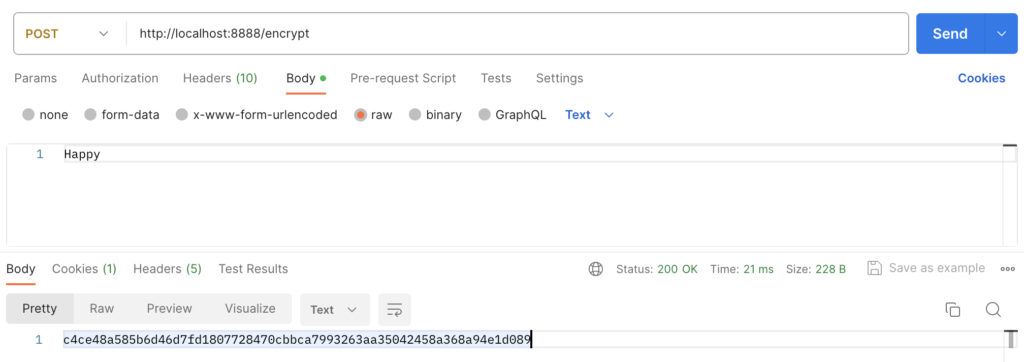
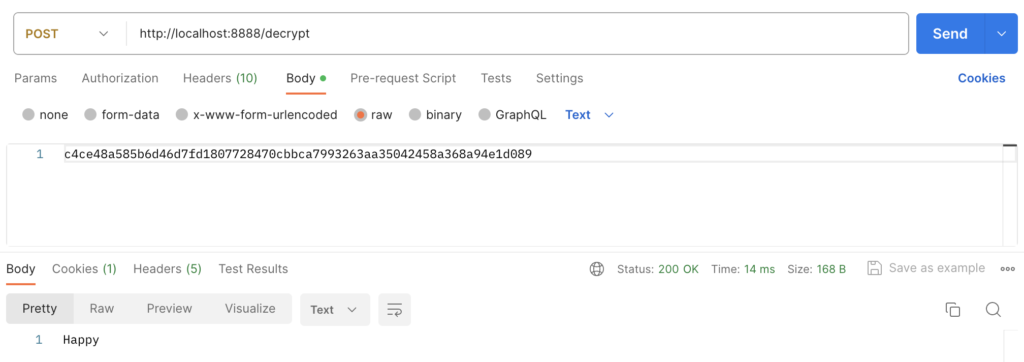
How to encrypt the data
You can run curl curl -X POST http://{config-server-host}:{port}/encrypt -d 'your_sensitive_data'
and place the encrypted value in configuration file with {cipher} prefix sensitive.property={cipher}encrypted_value_here
Decrypt on Client side
The setting spring.cloud.config.server.encrypt.enabled
to “true” on the Config Server would result in the server decrypting the data before transmitting it to the client. This configuration isn’t recommended for sensitive information, as it indeed defeats the purpose of encryption, so we need to keep this configuration disabled (‘false’)
spring.cloud.config.server.encrypt.enabled=false
On the client side, we need to switch this config to true
spring.cloud.config.server.encrypt.enabled=true
Spring Security
Currently, we’ve addressed security concerns from both Github and the http://localhost:8888/application/local
ends. However, there remains a vulnerability. The /encrypt
and /decrypt
endpoints are accessible, potentially allowing individuals to input encrypted data with decryption, revealing sensitive information. To mitigate this risk, we need to implement Spring Security to restrict access to these endpoints, ensuring they’re only available to authorized users.
Add Spring Security dependency.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId
</dependency>
And add these two configurations
spring.security.user.name=
spring.security.user.password=
Conclusion
The whole settings for Spring-Cloud-Config Server
dependency
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
ConfigServerApplication.java
@SpringBootApplication
@EnableConfigServer
public class ConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigServerApplication.class, args);
}
}
application.properties
##################
# Config Server
##################
spring.cloud.config.server.git.uri=
spring.cloud.config.server.git.username=
spring.cloud.config.server.git.password=
spring.cloud.config.server.git.default-label=main
management.endpoints.web.exposure.include=*
encrypt.key=
spring.cloud.config.server.encrypt.enabled=false
spring.security.user.name=
spring.security.user.password=
The whole settings for Spring-Cloud-Config Client
dependency
<dependency>
<groupId>org.springframework.cloud</groupId
<artifactId>spring-cloud-starter-config</artifactId>
<version>4.0.3</version>
</dependency>
application.properties
spring.profiles.active=local
spring.config.import=optional:configserver:http://localhost:8888/
management.endpoints.web.exposure.include=*
encrypt.key=
spring.cloud.config.server.encrypt.enabled=true