each entity class must have only one version attribute
it must be placed in the primary table for an entity mapped to several tables
type of a version attribute must be one of the following: int, Integer, long, Long, short, Short, java.sql.Timestamp
We should know that we can retrieve a value of the version attribute via entity, but we mustn’t update or increment it. Only the persistence provider can do that, so data stays consistent.
- What is the annotation in database like
- How it works
What is the annotation in database like
Example
Employee.java
@Data
@NoArgsConstructor
@Entity
public class Employee {
@Id
@GeneratedValue
private Integer id;
@Version
@Column(name="version")
private long version;
private String name;
private String department;
Employee(String name, String department) {
this.name = name;
this.department = department;
}
}
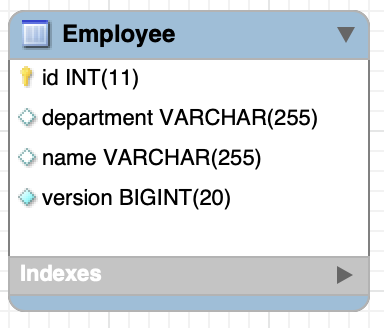
Demo11Application.java
@SpringBootApplication(exclude = SecurityAutoConfiguration.class)
public class Demo11Application {
public static void nativeQuery(EntityManager em, String s) {
System.out.printf("---------------------------%n'%s'%n", s);
Query query = em.createNativeQuery(s);
List list = query.getResultList();
for (Object o : list) {
if (o.getClass().isArray()) {
System.out.println(Arrays.toString((Object []) o));
}
}
}
public static void main(String[] args) {
EntityManagerFactory emf = Persistence.createEntityManagerFactory("PERSISTENCE1");
EntityManager em = emf.createEntityManager();
nativeQuery(em, "SHOW TABLES");
nativeQuery(em, "SHOW COLUMNS from Employee");
emf.close();
em.close();
// SpringApplication.run(Demo11Application.class, args);
}
}
How it works
Demo11Application.java
@SpringBootApplication(exclude = SecurityAutoConfiguration.class)
public class Demo11Application {
private static EntityManagerFactory entityManagerFactory =
Persistence.createEntityManagerFactory("PERSISTENCE1");
private static void updateEmployeeDepartment(String department, Object primaryKey) {
EntityManager em = entityManagerFactory.createEntityManager();
Employee employee = em.find(Employee.class, primaryKey);
em.getTransaction().begin();
employee.setDepartment(department);
em.getTransaction().commit();
em.close();
System.out.println("Employee updated: " + employee);
}
public static void persistEmployee() {
Employee employee = new Employee("Joe", "IT");
EntityManager em = entityManagerFactory.createEntityManager();
em.getTransaction().begin();
em.persist(employee);
em.getTransaction().commit();
em.close();
System.out.println("Employee persisted: " + employee);
}
public static void main(String[] args) {
try {
persistEmployee();
updateEmployeeDepartment("Sales", 1);
updateEmployeeDepartment("Admin", 1);
} finally {
entityManagerFactory.close();
}
// SpringApplication.run(Demo11Application.class, args);
}
}
