.fontWeight(.bold)
.font(.title) // built-in font size
.font(.system(size: 20)) // customized font size
.font(.custom("Helvetica Neue", size: 25))
.foregroundColor(.white)
.multilineTextAlignment(.center)
.lineSpacing(10)
.lineLimit(nil)
.truncationMode(.head)
.padding()
.rotationEffect(.degrees(45))
.rotationEffect(.degrees(20), anchor: UnitPoint(x: 0, y: 0))
.rotation3DEffect(.degrees(60), axis: (x: 1, y: 0, z: 0))
.shadow(color: .gray, radius: 2, x: 0, y: 15)
Example 2: sometimes you may feel .multilineTextAlignment(.center)
is not working. If you apply background(Color.blue), you may find that the frame for the text is pretty tight. In this case, we need enlarge the frame size.
GeometryReader{ geometry in
Text("goood")
.multilineTextAlignment(.trailing)
.frame(width: geometry.size.width, height: 100, alignment: .center)
.background(Color.blue)
}
System built-in fonts
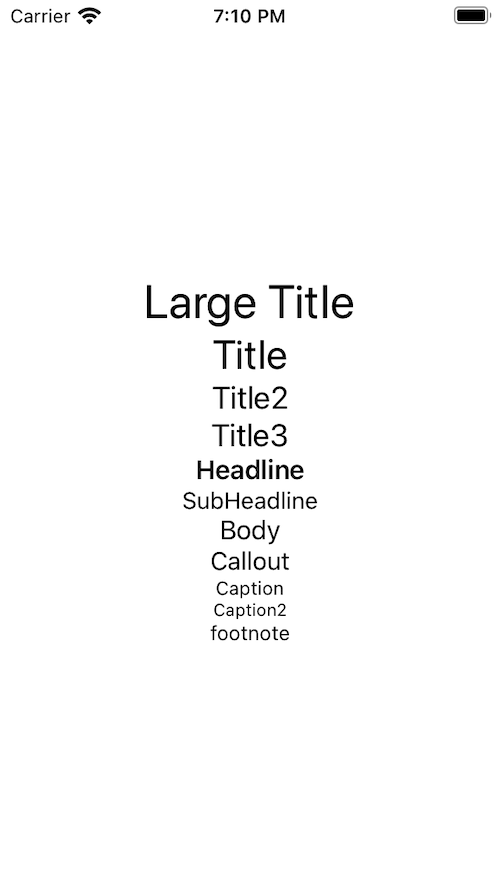
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
ZStack {
Text("largeTitle")
.font(.largeTitle)
Text("largeTitle")
.font(Font.system(size: 35))
}
ZStack {
Text("title")
.font(.title)
Text("title")
.font(Font.system(size: 30))
}
ZStack {
Text("title2")
.font(.title2)
Text("title2")
.font(Font.system(size: 23))
}
ZStack {
Text("title3")
.font(.title3)
Text("title3")
.font(Font.system(size: 20))
}
ZStack {
Text("body")
.font(.body)
Text("body")
.font(Font.system(size: 18))
}
ZStack {
Text("headline")
.font(.headline)
Text("headline")
.font(Font.system(size: 17))
.bold()
}
ZStack {
Text("subheadline")
.font(.subheadline)
Text("subheadline")
.font(Font.system(size: 15))
}
ZStack {
Text("callout")
.font(.callout)
Text("callout")
.font(Font.system(size: 15))
}
ZStack {
Text("footnote")
.font(.footnote)
Text("footnote")
.font(Font.system(size: 13))
}
ZStack {
Text("caption")
.font(.caption)
Text("caption")
.font(Font.system(size: 12))
}
}
ZStack {
Text("caption2")
.font(.caption2)
Text("caption2")
.font(Font.system(size: 10))
}
}
}